Java Overloading
package pace01;import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
import javax.swing.JOptionPane;
public class DateSixthTry
{
private String month;
private int day;
private int year; //a four digit number.
public void setDate(int monthInt, int day, int year)
{
if (dateOK(monthInt, day, year))
{
this.month = monthString(monthInt);
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(String monthString, int day, int year)
{
if (dateOK(monthString, day, year))
{
this.month = monthString;
this.day = day;
this.year = year;
}
else
{
System.out.println("Fatal Error");
System.exit(0);
}
}
public void setDate(int year)
{
setDate(1, 1, year);
}
private boolean dateOK(int monthInt, int dayInt, int yearInt)
{
return ( (monthInt >= 1) && (monthInt <= 12) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999) );
}
private boolean dateOK(String monthString, int dayInt, int yearInt)
{
return ( monthOK(monthString) &&
(dayInt >= 1) && (dayInt <= 31) &&
(yearInt >= 1000) && (yearInt <= 9999) );
}
private boolean monthOK(String month)
{
return (month.equals("January") || month.equals("February") ||
month.equals("March") || month.equals("April") ||
month.equals("May") || month.equals("June") ||
month.equals("July") || month.equals("August") ||
month.equals("September") || month.equals("October") ||
month.equals("November") || month.equals("December") );
}
public void readInput( )
{
boolean tryAgain = true;
String keyboard=JOptionPane.showInputDialog("Enter keybord");
while (tryAgain)
{
System.out.println("Enter month, day, and year.");
System.out.println("Do not use a comma.");
String monthInput = keyboard;
int dayInput = Integer.parseInt(keyboard);
int yearInput = Integer.parseInt(keyboard);
if (dateOK(monthInput, dayInput, yearInput) )
{
setDate(monthInput, dayInput, yearInput);
tryAgain = false;
}
else
System.out.println("Illegal date. Reenter input.");
}
}
public void writeOutput( )
{
System.out.println(month + " " + day + ", " + year);
}
public void setMonth(int monthNumber)
{
if ((monthNumber <= 0) || (monthNumber > 12))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
month = monthString(monthNumber);
}
public void setDay(int day)
{
if ((day <= 0) || (day > 31))
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.day = day;
}
public void setYear(int year)
{
if ( (year < 1000) || (year > 9999) )
{
System.out.println("Fatal Error");
System.exit(0);
}
else
this.year = year;
}
public boolean equals(DateSixthTry otherDate)
{
return ( (month.equalsIgnoreCase(otherDate.month))
&& (day == otherDate.day) && (year == otherDate.year) );
}
public boolean precedes(DateSixthTry otherDate)
{
return ( (year < otherDate.year) ||
(year == otherDate.year && getMonth( ) < otherDate.getMonth( )) ||
(year == otherDate.year && month.equals(otherDate.month)
&& day < otherDate.day) );
}
public String toString( )
{
return (month + " " + day + ", " + year);
}
public int getDay( )
{
return day;
}
public int getYear( )
{
return year;
}
public int getMonth( )
{
if (month.equalsIgnoreCase("January"))
return 1;
else if (month.equalsIgnoreCase("February"))
return 2;
else if (month.equalsIgnoreCase("March"))
return 3;
else if (month.equalsIgnoreCase("April"))
return 4;
else if (month.equalsIgnoreCase("May"))
return 5;
else if (month.equals("June"))
return 6;
else if (month.equalsIgnoreCase("July"))
return 7;
else if (month.equalsIgnoreCase("August"))
return 8;
else if (month.equalsIgnoreCase("September"))
return 9;
else if (month.equalsIgnoreCase("October"))
return 10;
else if (month.equalsIgnoreCase("November"))
return 11;
else if (month.equalsIgnoreCase("December"))
return 12;
else
{
System.out.println("Fatal Error");
System.exit(0);
return 0; //Needed to keep the compiler happy
}
}
private String monthString(int monthNumber)
{
switch (monthNumber)
{
case 1:
return "January";
case 2:
return "February";
case 3:
return "March";
case 4:
return "April";
case 5:
return "May";
case 6:
return "June";
case 7:
return "July";
case 8:
return "August";
case 9:
return "September";
case 10:
return "October";
case 11:
return "November";
case 12:
return "December";
default:
System.out.println("Fatal Error");
System.exit(0);
return "Error"; //to keep the compiler happy
}
}
public void setMonth(String month){ int monthInt;
if (month=="January")
{ monthInt= 1;}
else if (month=="February")
{ monthInt= 2;}
else if (month=="March")
{ monthInt= 3;}
else if (month=="April")
{ monthInt= 4;}
else if (month=="May")
{monthInt= 5;}
else if(month=="June")
{ monthInt= 6;}
else if (month=="July")
{ monthInt= 7;}
else if (month=="August")
{ monthInt= 8;}
else if (month=="September")
{ monthInt= 9;}
else if (month=="October")
{ monthInt= 10;}
else if (month=="November")
{ monthInt= 11;}
else if (month=="December")
{ monthInt= 12;}
else
{
System.out.println("Fatal Error");
System.exit(0);
{ monthInt= 0;} //Needed to keep the compiler happy
}
this.setMonth(monthInt);
}}
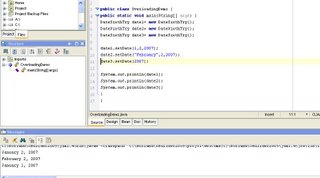
0 Comments:
Post a Comment
<< Home